#!/bin/sh # # Goran Cvetanoski - 19/12/2006 # # pwage # # This script works out the time left before a password expires # # It will send a reminder email 10 days and 3 days before the password # will expire. The email will go to unix.admin@mydomain.com.au unless an # alternate email address is specified. An email will also be sent if a # password has expired. # # The following command will send results to unix.admin@mydomain.com.au # pwage oracle # # Specify an alternate email address if you would like the results to be # sent to a different email address. # ie: # pwage oracle oracledba@mydomain.com.au # # # CHANGE LOG # ========================================================================= # 19/12/2006 - Goran Base script created # 05/08/2009 - Ricky Smith added code to check each user # LOG=/tmp/pwage.log DASHES="-----------------------------" show() { echo "$DASHES $1 $DASHES" >> $LOG shift eval "$@" >> $LOG echo "" >> $LOG } SendMail() { cat $LOG | mailx -s "$1" "$2" } reminder () { echo "Date: `date`" echo "" echo "Please change your password within the next $EXPIRE days" } expired () { echo "Date: `date`" echo "" echo "The password for $USER has expired" echo "$USER last changed their password on $LSTCNG" echo "The maximum age for the password is $MAX days" echo "and it has expired $EXPIRE days ago" } CheckUser() { USER=$1 EMAIL=$2 CURRENT_EPOCH=`grep $USER /etc/shadow | cut -d: -f3` if [ "$CURRENT_EPOCH" = "" ]; then return fi # Find the epoch time since the user's password was last changed EPOCH=`perl -e 'print int(time/(60*60*24))'` # Compute the age of the user's password AGE=`echo $EPOCH - $CURRENT_EPOCH | bc` # Compute and display the number of days until password expiration MAX=`grep $USER /etc/shadow | cut -d: -f5` if [ "$MAX" = "" ]; then return fi EXPIRE=`echo $MAX - $AGE | bc` CHANGE=`echo $CURRENT_EPOCH + 1 | bc` LSTCNG="`perl -e 'print scalar localtime('$CHANGE' * 24 *3600);'`" WARN=`grep $USER /etc/shadow | cut -d: -f6` if [ "$WARN" = "" ]; then WARN=0 fi if [ "$EXPIRE" -le "$WARN" ]; then show "R E M I N D E R" reminder SendMail "$USER Password Info On `uname -n`" "$EMAIL" elif [ "$EXPIRE" -lt 0 ]; then show "E X P I R E D" expired SendMail "WARNING: $USER Password Expired On `uname -n`" "$EMAIL" fi } # Main Code domain=$1 if [ "$domain" = "" ]; then domain=$(dnsdomainname) fi minuid=$2 if [ "$minuid" = "" ]; then minuid=500 fi IFS=':' while read user pass uid gid full home shell do if [ $uid -ge $minuid ]; then cat /dev/null > $LOG CheckUser $user "\"$full\" <$user@$domain>" fi done </etc/passwd
010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101010101
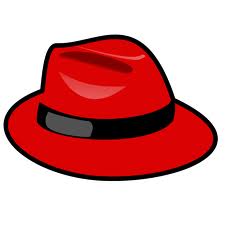
Tuesday, 13 September 2011
password expired mail alert script:
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment